Overview The General Data Protection Regulation (GDPR) requires websites to protect their users' data. If…
Add Custom Payment Gateway in WooCommerce Easily
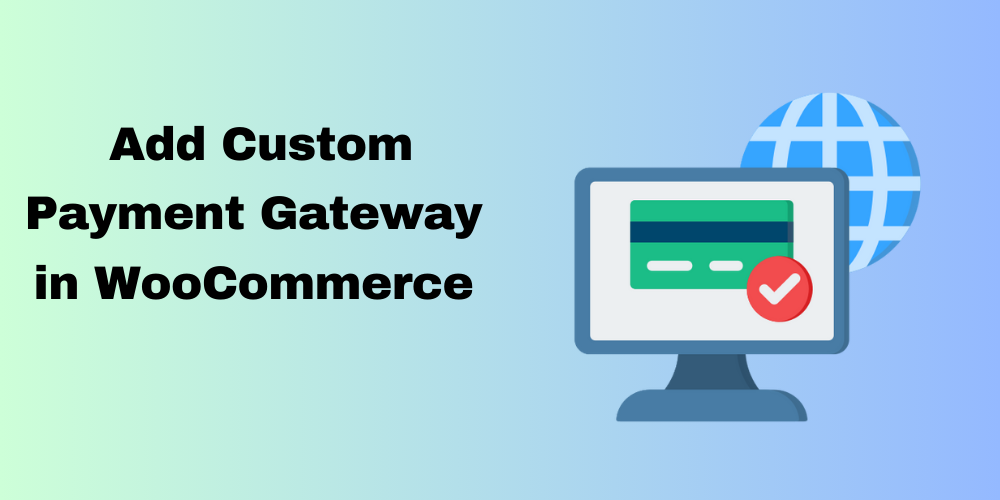
Overview
When you’re building a WooCommerce store, the default payment gateways might not always meet your specific business requirements. If you need to implement a custom payment gateway in WooCommerce, you’re in the right place. In this guide, we’ll walk you through the process of creating a custom payment method in WooCommerce, so you can offer your customers a more personalized payment experience.
Why Add a Custom Payment Gateway in WooCommerce?
While WooCommerce provides a range of default payment methods, sometimes you might need a custom payment solution. Here are a few reasons to add a custom payment method in WooCommerce:
- Business-Specific Needs: Certain businesses have unique payment workflows, such as recurring subscriptions or region-specific payment options.
- Third-Party Integration: If you’re integrating with a third-party service that doesn’t offer a WooCommerce plugin, creating a custom gateway is the solution.
- Cost and Flexibility: You can control the fees, terms, and how the payments are processed, offering better terms for your business.
Step-by-Step Guide to Add a Custom Payment Gateway in WooCommerce
The following code snippet will guide you through adding a custom payment gateway to WooCommerce. It’s a simple yet effective solution to extend your WooCommerce store’s payment options.
1. Create a Custom Payment Gateway Class
You need to create a class that defines the custom payment gateway. This class will handle all of the logic for processing payments.
add_action('plugins_loaded', 'add_custom_gateway');
function add_custom_gateway() {
class WC_Gateway_Custom extends WC_Payment_Gateway {
public function __construct() {
$this->id = 'custom';
$this->method_title = 'Custom Gateway';
// Additional setup such as description and other configurations
}
public function process_payment($order_id) {
$order = wc_get_order($order_id);
// Your payment processing logic here
// Example: Mark the order as processing and return a success response
$order->update_status('processing');
return array('result' => 'success', 'redirect' => $this->get_return_url($order));
}
}
// Add custom payment method to WooCommerce
add_filter('woocommerce_payment_gateways', 'add_custom_gateway_to_list');
function add_custom_gateway_to_list($methods) {
$methods[] = 'WC_Gateway_Custom';
return $methods;
}
}
2. Configure Your Custom Payment Gateway
In the constructor of the WC_Gateway_Custom
class, you can set up various configurations like the title, description, instructions, and even the icon for the custom payment method.
Example configuration:
$this->method_title = 'Custom Payment Gateway';
$this->method_description = 'Use this payment method for custom transactions';
$this->title = 'Custom Gateway';
This allows you to customize how the payment gateway appears in the WooCommerce checkout process.
3. Payment Processing Logic
In the process_payment()
method, you will need to define the steps for handling the payment. This might include integrating an external API, verifying transaction status, or processing payments directly.
Example:
public function process_payment($order_id) {
$order = wc_get_order($order_id);
// Process the payment logic
// Example: Call external payment gateway API to process the payment
if ($payment_success) {
$order->update_status('completed');
return array('result' => 'success', 'redirect' => $this->get_return_url($order));
} else {
return array('result' => 'failure', 'message' => 'Payment failed.');
}
}
4. Adding Your Custom Payment Gateway to WooCommerce
To make your custom payment gateway for WooCommerce visible in the WooCommerce checkout page, we use the woocommerce_payment_gateways
filter. This adds your custom gateway to the list of available payment options.
Testing Your Custom Payment Gateway
Once you’ve added your custom payment method to WooCommerce, it’s time to test it. Here are some things to verify:
- Ensure the payment gateway shows up on the WooCommerce checkout page.
- Test the payment processing functionality with sandbox or test credentials.
- Check if the order status updates correctly after payment processing.
Conclusion
By following this guide, you can successfully add a custom payment method in WooCommerce that fits your store’s needs. Whether you need a unique payment option for your customers or you’re integrating a third-party solution, custom payment gateways for WooCommerce provide the flexibility to tailor the payment process to your business.
With this WooCommerce custom payment gateway, you can enhance the user experience and streamline the checkout process for your customers, ensuring they have more payment options suited to their preferences.
FAQ
How Do I Add a Custom Payment Gateway to WooCommerce?
You can add a custom payment gateway by creating a custom class that extends WC_Payment_Gateway
, adding your payment logic, and registering it with WooCommerce via the woocommerce_payment_gateways
filter.
Can I Use My Own API with a Custom Payment Gateway?
Yes, you can integrate any third-party payment gateway API within the process_payment()
method of your custom class.
How Can I Test My Custom Payment Gateway?
You should use sandbox/test credentials provided by the payment service provider and run test transactions to ensure the payment logic works correctly.
This Post Has 0 Comments