Overview The General Data Protection Regulation (GDPR) requires websites to protect their users' data. If…
How to Use the WordPress REST API: A Complete Guide
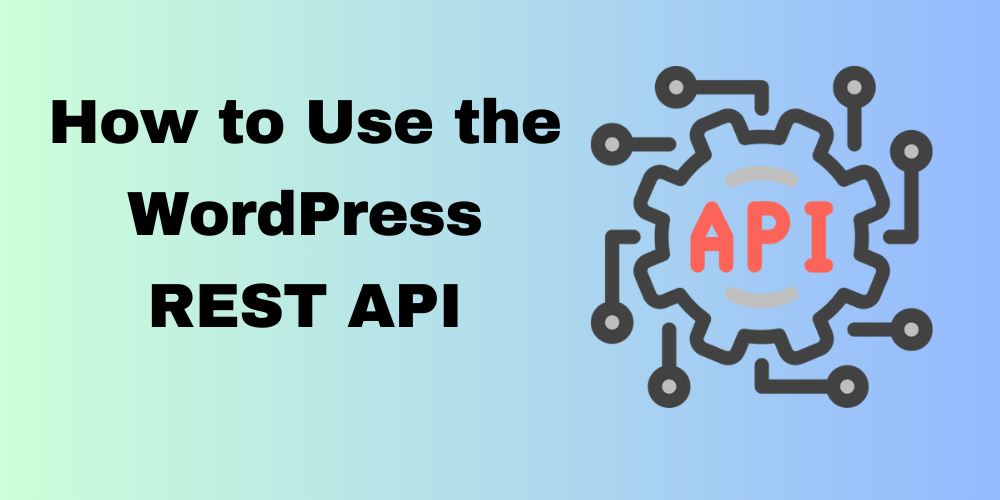
Overview
The WordPress REST API is a powerful tool that allows developers to interact with their WordPress site programmatically. Whether you’re building a mobile app, a single-page application (SPA), or integrating with other services, the WordPress API offers a flexible way to manage WordPress content without needing to log in to the WordPress dashboard.
In this guide, we’ll walk you through the basics of using the WordPress REST API, including how to fetch data, create new content, secure your API endpoints, and extend the REST API with WordPress.
What is the WordPress REST API?
The WordPress REST API enables communication between your WordPress site and other software or applications. It allows you to retrieve, create, update, and delete data on your site through HTTP requests. This means that you can integrate WordPress with the REST API, automate workflows, or build dynamic, interactive websites and apps without directly interacting with the WordPress admin panel.
By default, the REST API in WordPress is enabled in WordPress 4.7 and later, making it easy to start integrating WordPress with external systems or building custom features.
Why Use the WordPress REST API?
By leveraging the WordPress REST API, you can build a more dynamic and interactive website or integrate WordPress seamlessly with other services. It’s a powerful tool for developers looking to expand WordPress functionality beyond the traditional dashboard interface.
Whether you’re building an app, automating processes, or just want more control over your site’s data, the REST API provides a flexible, secure, and extensible way to work with your WordPress content.
Steps to Use the WordPress REST API
1. Enable the REST API
The WP REST API is automatically enabled in WordPress 4.7 and later. You don’t need to do anything special to activate it. You can access the API by visiting this URL format:
https://yourwebsite.com/wp-json/wp/v2/
This URL provides access to all available API routes. From here, you can query your site for posts, pages, custom post types, and much more.
2. Fetching Data from WordPress Using the WP JSON API
o retrieve content from your WordPress site, you can use JavaScript’s fetch()
function or a library like Axios. For example, to fetch all posts from your WordPress site, use this simple fetch() code:
fetch('https://yourwebsite.com/wp-json/wp/v2/posts')
.then(response => response.json())
.then(data => console.log(data));
This will return an array of all your posts in JSON format, which you can then use in your application.
3. Creating New Content
The REST API also allows you to create new content, such as posts, directly from external applications. To create a new post, you’ll need to send a POST
request to the following endpoint:
POST https://yourwebsite.com/wp-json/wp/v2/posts
Here’s an example of how to create a new post:
fetch('https://yourwebsite.com/wp-json/wp/v2/posts', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': 'Bearer YOUR_ACCESS_TOKEN'
},
body: JSON.stringify({
title: 'New Post Title',
content: 'This is the content of the new post.',
status: 'publish'
})
})
.then(response => response.json())
.then(data => console.log('New post created:', data));
This request will create a new post with the specified title and content.
4. Securing the API
For security reasons, it’s important to secure your REST API endpoints to prevent unauthorized access. You can use several authentication methods:
- OAuth Authentication
- Application Passwords
- Custom Authentication
Here’s a quick example using Bearer Token Authentication:
'Authorization': 'Bearer YOUR_ACCESS_TOKEN'
Make sure to store your API keys securely and avoid exposing them in client-side code.
5. Extending the REST API
You can also extend the WordPress REST API by adding custom endpoints to meet your specific needs. For example, if you want to create an endpoint to return custom data, you can register a new route like this:
add_action('rest_api_init', function () {
register_rest_route('custom/v1', '/data/', array(
'methods' => 'GET',
'callback' => 'get_custom_data',
));
});
function get_custom_data() {
return new WP_REST_Response(array('data' => 'This is custom data'), 200);
}
This will allow you to fetch custom data by visiting:
https://yourwebsite.com/wp-json/custom/v1/data/
Conclusion
The WordPress REST API opens up a world of possibilities for developers. By following the steps in this guide, you’ll be able to fetch data, create content, secure your API endpoints, and extend the functionality of your WordPress site.
Are you ready to start using the WordPress REST API in your next project? Share your thoughts in the comments below!
This Post Has 0 Comments