Learn the difference between free and premium WordPress themes. Find out which one is right for your website with pros, cons, and tips.
How to Easily Implementing wordpress ajax pagination without plugin
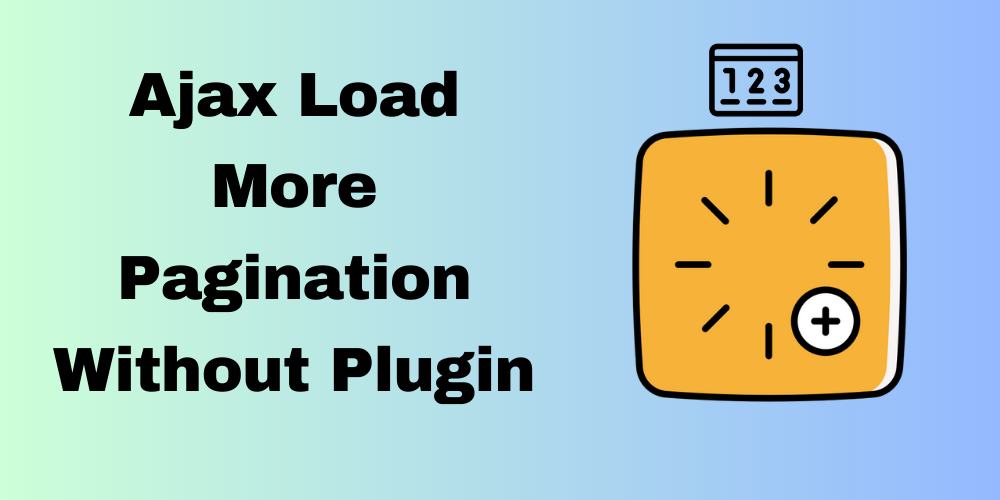
Table of Contents
- Overview
- What is AJAX in WordPress?
- Set Up the Environment
- Method 1: Load More From Page 2
A. Template Code
B. Custom Page Template - Method 2: Load More From Page 1
- Enqueue JavaScript in
functions.php
- Backend Function to Load Posts
- Support for Custom Post Types or Category Filter
- Custom Post Types or Category Filter Support
- Conclusion
- FAQ
Overview
In web development, creating smooth user experiences is crucial. Users often expect seamless navigation, especially in blogs with extensive content. Traditional pagination can disrupt this flow by reloading the entire page. However, using AJAX (Asynchronous JavaScript and XML), we can implement a “Load More” button. This feature allows users to fetch additional content without refreshing the page. In this blog post, we will demonstrate how to achieve this in WordPress, focusing on custom post types and regular blog posts.
Understanding AJAX in WordPress:
WordPress has robust support for AJAX through the admin-ajax.php file. This allows developers to handle AJAX requests effectively. By using action hooks, developers can run custom PHP functions without reloading the page. This process enhances user experience significantly.
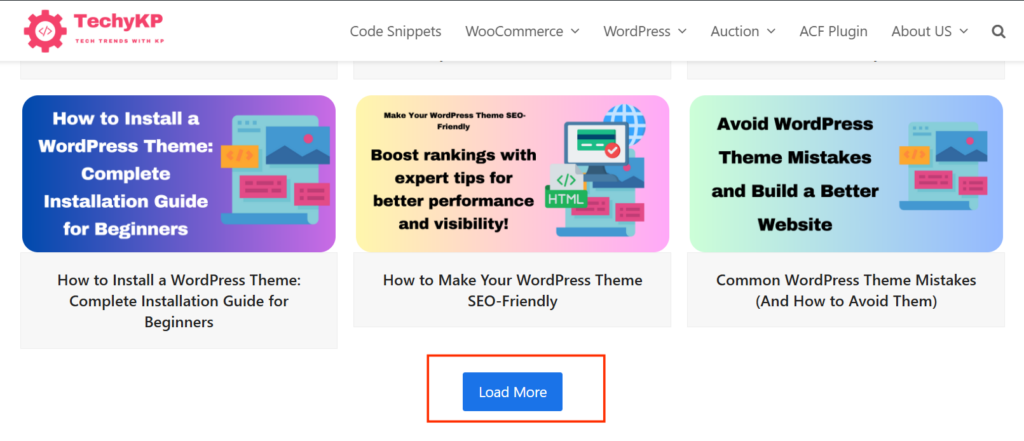
Set Up the Environment
Create a load-more.js
file in your theme folder:
jQuery(document).ready(function($) {
var page = 2;
$('.loadmore').on('click', function() {
var data = {
'action': 'codebykishor_load_more_posts',
'page': page,
'post_type': loadmore_params.post_type,
'category': loadmore_params.category
};
$.post(loadmore_params.ajaxurl, data, function(response) {
$('.posts').append(response);
page++;
});
});
// Optional: Load first page automatically (Method 2 only)
if ($('.posts').is(':empty')) {
$('.loadmore').click();
}
});
Method 1: Load More from Page 2
Option 1: Template Code for index.php
or archive.php
If you’re adding the functionality to your main blog page or an archive:
<div class="posts">
<?php
$args = array(
'post_type' => 'post',
'posts_per_page' => 5,
'paged' => 1
);
$query = new WP_Query($args);
if ($query->have_posts()) :
while ($query->have_posts()) : $query->the_post();
echo '<h2>' . get_the_title() . '</h2>';
endwhile;
wp_reset_postdata();
endif;
?>
</div>
<button class="loadmore">Load More</button>
Option 2: Custom Page Template (e.g. page-ajax-loadmore.php
)
Create a new file in your theme folder called page-ajax-loadmore.php
and add this:
<?php
/*
Template Name: AJAX Load More (Page 2 Start)
*/
get_header();
?>
<div class="container">
<div class="posts">
<?php
$args = array(
'post_type' => 'post',
'posts_per_page' => 5,
'paged' => 1
);
$query = new WP_Query($args);
if ($query->have_posts()) :
while ($query->have_posts()) : $query->the_post();
echo '<h2>' . get_the_title() . '</h2>';
endwhile;
wp_reset_postdata();
endif;
?>
</div>
<button class="loadmore">Load More</button>
</div>
<?php get_footer(); ?>
Steps to Use the Template
- Go to Pages > Add New in your WordPress dashboard.
- Name it something like Blog AJAX Page.
- On the right sidebar under Template, select AJAX Load More (Page 2 Start).
- Publish the page.
Method 2: Load More from Page 1
Template (e.g. page-ajax.php
):
<div class="posts"></div>
<button class="loadmore">Load More</button>
This method uses AJAX to load even the first page.
Enqueue JavaScript in functions.php
function enqueue_load_more_script() {
wp_enqueue_script(
'load-more-script',
get_stylesheet_directory_uri() . '/js/load-more.js',
array('jquery'),
null,
true
);
wp_localize_script('load-more-script', 'loadmore_params', array(
'ajaxurl' => admin_url('admin-ajax.php'),
'post_type' => get_post_type(), // dynamic post type
'category' => get_queried_object()->slug ?? ''
));
}
add_action('wp_enqueue_scripts', 'enqueue_load_more_script');
Backend Function to Load Posts (Paste in functions.php
)
function codebykishor_load_more_posts() {
$paged = isset($_POST['page']) ? intval($_POST['page']) : 1;
$post_type = sanitize_text_field($_POST['post_type'] ?? 'post');
$category = sanitize_text_field($_POST['category'] ?? '');
$args = array(
'post_type' => $post_type,
'posts_per_page' => 5,
'paged' => $paged,
);
if ($category && $post_type === 'post') {
$args['category_name'] = $category;
}
$query = new WP_Query($args);
if ($query->have_posts()) :
while ($query->have_posts()) : $query->the_post();
echo '<h2>' . get_the_title() . '</h2>';
endwhile;
else :
echo '<p>No more posts found.</p>';
endif;
wp_reset_postdata();
wp_die();
}
add_action('wp_ajax_codebykishor_load_more_posts', 'codebykishor_load_more_posts');
add_action('wp_ajax_nopriv_codebykishor_load_more_posts', 'codebykishor_load_more_posts');
Support for Custom Post Types
Just change the post_type
in your template:
// For example, for a custom post type "portfolio"
wp_localize_script('load-more-script', 'loadmore_params', array(
'ajaxurl' => admin_url('admin-ajax.php'),
'post_type' => 'portfolio', // ← Your custom post type
'category' => ''
));
And you can style or format the output inside the PHP loop however you want!
Support for Category Filtering
To filter by category, pass the category slug in wp_localize_script()
like this:
'category' => get_queried_object()->slug ?? ''
This way, your AJAX query will only fetch posts from that specific category.
Custom Post Types or Category Filter Support
To show posts from a custom post type or specific category, change the WP_Query
parameters in both the template and AJAX function:
Template (Example: Custom Post Type portfolio
with Category design
)
$args = array(
'post_type' => 'portfolio',
'posts_per_page' => 5,
'paged' => 1,
'tax_query' => array(
array(
'taxonomy' => 'portfolio_category',
'field' => 'slug',
'terms' => 'design'
)
)
);
AJAX Handler
function codebykishor_load_more_posts() {
$paged = $_POST['page'];
$args = array(
'post_type' => 'portfolio',
'posts_per_page' => 5,
'paged' => $paged,
'tax_query' => array(
array(
'taxonomy' => 'portfolio_category',
'field' => 'slug',
'terms' => 'design'
)
)
);
$query = new WP_Query($args);
if ($query->have_posts()) :
while ($query->have_posts()) : $query->the_post();
echo '<h2>' . get_the_title() . '</h2>';
endwhile;
endif;
wp_die();
}
add_action('wp_ajax_codebykishor_load_more_posts', 'codebykishor_load_more_posts');
add_action('wp_ajax_nopriv_codebykishor_load_more_posts', 'codebykishor_load_more_posts');
Enqueue JavaScript and Handle AJAX
In your theme’s functions.php
:
function enqueue_load_more_script() {
wp_enqueue_script('load-more-script', get_stylesheet_directory_uri() . '/js/load-more.js', array('jquery'), '1.0', true);
wp_localize_script('load-more-script', 'loadmore_params', array(
'ajaxurl' => admin_url('admin-ajax.php')
));
}
add_action('wp_enqueue_scripts', 'enqueue_load_more_script');
Conclusion
You now have two methods to load more posts using AJAX:
- Method 1: Starts from page 2
- Method 2: Starts from page 1 (fully AJAX)
And you also know how to add:
- Custom post type support
- Category filtering
No plugins. Just clean code and smooth performance!
FAQ
What is AJAX?
AJAX stands for Asynchronous JavaScript and XML. It allows web pages to update asynchronously by exchanging small amounts of data with the server behind the scenes.
How does the “Load More” button work?
When users click the “Load More” button, an AJAX request is sent to the server to fetch additional posts. These posts are then appended to the existing content without refreshing the page.
Do I need to write any custom code?
Yes, you need to write custom PHP and JavaScript code to implement the AJAX functionality in WordPress.
Can I customize the number of posts displayed?
Absolutely! You can adjust the posts_per_page
parameter in the custom query to display any number of posts you prefer.
This Post Has 0 Comments