Learn the difference between WordPress Cron Jobs and Server Cron Jobs with examples, pros and cons. Make sure your website tasks run smoothly.
How to Create Admin Menu in WordPress and Add Menu Item
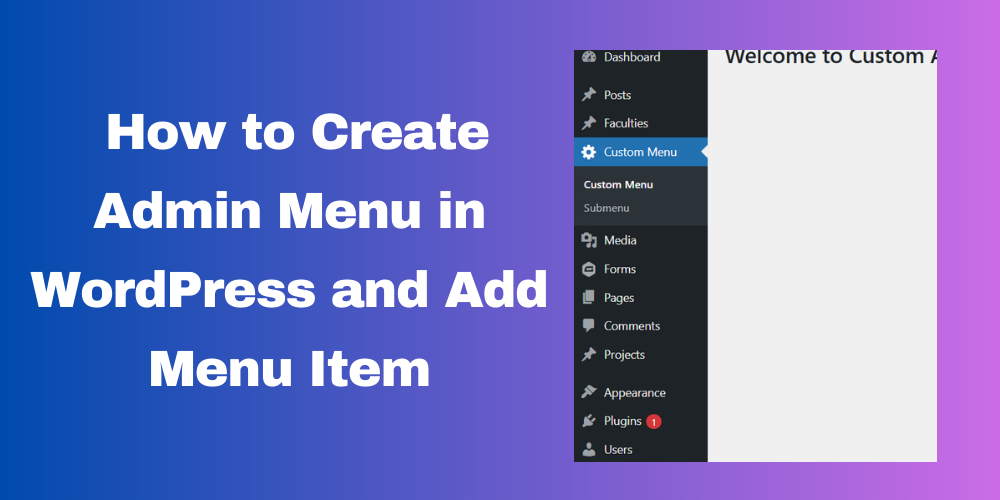
Table of Contents
- Introduction
- Why Add a Custom Admin Menu in WordPress?
- How to Create an Admin Menu in WordPress
- Adding Submenus to the Admin Menu
- Adding a Null Section to the Admin Menu
- Restricting Access to Admin Menus
- Full Code for Creating Admin Menus
- Conclusion
- FAQ
Introduction
WordPress allows developers to create custom admin menus to add new sections in the dashboard. In this guide, you will learn how to create an admin menu in WordPress and add a menu item.
Why Add a Custom Admin Menu in WordPress?
- Provides easy access to custom settings pages
- Enhances user experience by organizing features
- Helps add new functionalities for site admins
How to Create an Admin Menu in WordPress
To add an admin menu in WordPress, use the add_menu_page()
function.
Example Code
function custom_admin_menu() {
add_menu_page(
'Custom Menu Title', // Page Title
'Custom Menu', // Menu Title
'manage_options', // Capability
'custom-menu-slug', // Menu Slug
'custom_menu_page', // Callback Function
'dashicons-admin-generic', // Icon
6 // Position
);
}
add_action('admin_menu', 'custom_admin_menu');
function custom_menu_page() {
echo '<h1>Welcome to Custom Admin Page</h1>';
}
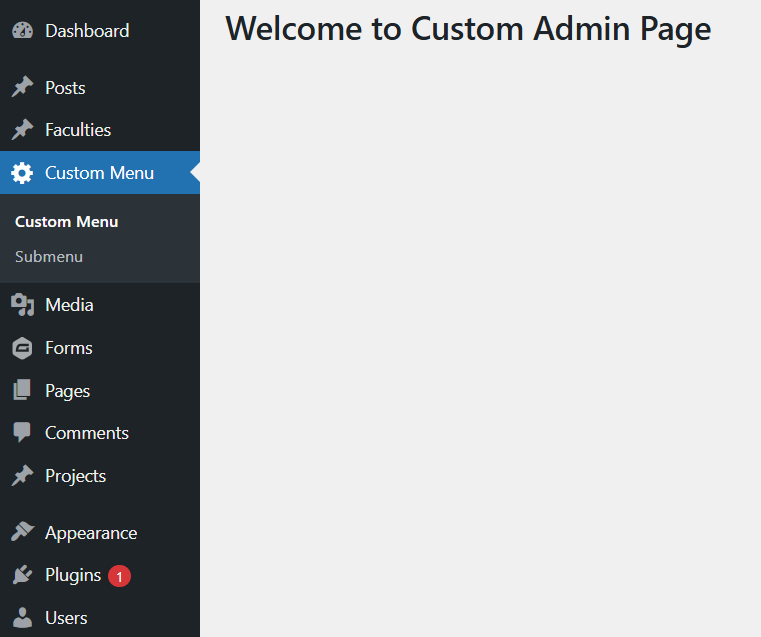
Adding Submenus to the Admin Menu
You can add submenus using add_submenu_page()
.
Example Code
function custom_submenu() {
add_submenu_page(
'custom-menu-slug', // Parent Slug
'Submenu Page Title', // Page Title
'Submenu', // Menu Title
'manage_options', // Capability
'custom-submenu-slug', // Menu Slug
'custom_submenu_page' // Callback Function
);
}
add_action('admin_menu', 'custom_submenu');
function custom_submenu_page() {
echo '<h1>Welcome to Custom Submenu Page</h1>';
}
Adding a Null Section to the Admin Menu
In some cases, you may want to add a submenu item without a parent menu. You can achieve this by using null
as the parent slug.
Example Code
function null_submenu_section() {
add_submenu_page(
'null', // No parent menu
'Null Section', // Page Title
'Null Section', // Menu Title
'manage_options', // Capability
'null-section-slug', // Menu Slug
'null_section_page' // Callback Function
);
}
add_action('admin_menu', 'null_submenu_section');
function null_section_page() {
echo '<h1>Welcome to Null Section</h1>';
}
Restricting Access to Admin Menus
To restrict access, use current_user_can()
to check user roles.
function custom_admin_menu_restricted() {
if (current_user_can('manage_options')) {
add_menu_page(
'Restricted Menu',
'Restricted',
'manage_options',
'restricted-menu-slug',
'restricted_menu_page',
'dashicons-lock',
7
);
}
}
add_action('admin_menu', 'custom_admin_menu_restricted');
function restricted_menu_page() {
echo '<h1>Restricted Access Page</h1>';
}
Full Code for Creating Admin Menus
Here is the complete PHP code that combines adding an admin menu, submenus, a null menu, and access restrictions into one file:
function custom_admin_menus() {
// Main Menu
add_menu_page(
'Custom Menu Title', // Page Title
'Custom Menu', // Menu Title
'manage_options', // Capability
'custom-menu-slug', // Menu Slug
'custom_menu_page', // Callback Function
'dashicons-admin-generic', // Icon
6 // Position
);
// Submenu
add_submenu_page(
'custom-menu-slug', // Parent Slug
'Submenu Page Title', // Page Title
'Submenu', // Menu Title
'manage_options', // Capability
'custom-submenu-slug', // Menu Slug
'custom_submenu_page' // Callback Function
);
// Null Section
add_submenu_page(
'null', // No parent menu
'Null Section', // Page Title
'Null Section', // Menu Title
'manage_options', // Capability
'null-section-slug', // Menu Slug
'null_section_page' // Callback Function
);
// Restricted Menu
if (current_user_can('manage_options')) {
add_menu_page(
'Restricted Menu',
'Restricted',
'manage_options',
'restricted-menu-slug',
'restricted_menu_page',
'dashicons-lock',
7
);
}
}
add_action('admin_menu', 'custom_admin_menus');
function custom_menu_page() {
echo '<h1>Welcome to Custom Admin Page</h1>';
}
function custom_submenu_page() {
echo '<h1>Welcome to Custom Submenu Page</h1>';
}
function null_section_page() {
echo '<h1>Welcome to Null Section</h1>';
}
function restricted_menu_page() {
echo '<h1>Restricted Access Page</h1>';
}
Conclusion
Creating an admin menu in WordPress is a simple way to add custom functionalities to the dashboard. You can also add submenus and restrict access based on user roles.
FAQ
Can I add a custom admin menu without a plugin?
Yes, you can add a custom admin menu using the add_menu_page()
function in your theme’s functions.php
file.
How do I change the admin menu icon?
Use the dashicons
class in the add_menu_page()
function. Example: 'dashicons-admin-generic'
.
Can I hide the admin menu for specific users?
Yes, use current_user_can()
to restrict access based on user roles.
This Post Has 0 Comments