Learn the difference between free and premium WordPress themes. Find out which one is right for your website with pros, cons, and tips.
The Ultimate Guide to API vs Webhook: Differences, Benefits, and When to Use
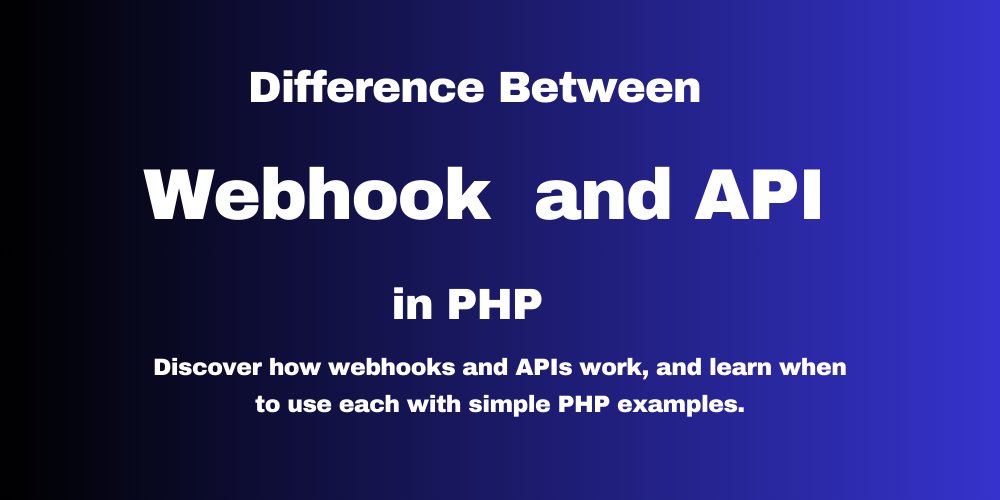
Webhooks and APIs both help software systems communicate, but they work in different ways. If you’re building or integrating apps, knowing when to use a webhook vs API can make your project more efficient.
Table of Contents
- What is an API?
- What is a Webhook?
- Webhook vs API: Key Differences
- PHP Examples: Webhook vs API
- When to Use an API vs Webhook
- FAQs
What is an API?
API stands for Application Programming Interface. It allows applications to request data or actions from other software. Think of an API like a waiter at a restaurant: it takes your order, brings it to the kitchen, and returns with what you asked for.
Examples of APIs:
- Find nearby restaurants using a Places API
- Display maps with a Mapping API
- Handle payments via Payment Gateway APIs
- Share content with Social Media APIs
APIs require you to send a request to get a response. You control when the communication happens.
What is a Webhook?
A webhook is an automated message sent from one app to another when a specific event happens. Unlike an API, you don’t have to ask for data—it’s pushed to you in real time.
Imagine your phone buzzes instantly when your favorite band releases a new song. That’s like a webhook sending you instant data.
How Webhooks Work:
- You provide a URL to receive updates.
- The service sends data when something happens.
- No request is needed from your side.
Webhooks are great for real-time notifications like form submissions, payment updates, or email events.
Webhook vs API: Key Differences
Feature | API | Webhook |
---|---|---|
Communication Direction | Request-response | Event-driven (server pushes updates) |
Data Transfer | Initiated by you | Initiated by the source |
Real-Time Updates | Not real-time (requires polling) | Real-time |
Resource Usage | Higher due to repeated requests | Lower (no polling required) |
Best Use Cases | Data lookup, create/update data | Notifications, instant updates |
PHP Examples: Webhook vs API
API Example (Using cURL to GET Data)
<?php
$apiUrl = "https://api.weatherapi.com/v1/current.json?key=YOUR_API_KEY&q=London";
$ch = curl_init($apiUrl);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
$data = json_decode($response, true);
echo "Current Temperature in London: " . $data['current']['temp_c'] . "°C";
?>
Webhook Example (Receiving POST Data)
<?php
$webhookData = file_get_contents("php://input");
$data = json_decode($webhookData, true);
file_put_contents("webhook-log.txt", print_r($data, true), FILE_APPEND);
echo json_encode(["status" => "received"]);
?>
When to Use an API vs Webhook
Use APIs when:
- You need to request data at specific times
- You want to create or update resources
- Real-time updates aren’t required
Use Webhooks when:
- You need real-time notifications
- You want to automate workflows
- You want to reduce server load
Often, combining both gives you the best of both worlds.
FAQs
Can I use both Webhooks and APIs together?
Yes! Many applications use APIs for regular data handling and webhooks for real-time alerts.
Are Webhooks faster than APIs?
Webhooks are faster for event-based updates since they push data instantly.
Is a webhook more secure than an API?
Both can be secure. Use authentication, HTTPS, and validation techniques for security.
What happens if my webhook URL is down?
The sending service may retry later or fail the delivery. Some platforms offer retry logic.
How do I test a webhook?
Use tools like RequestBin or Ngrok to simulate webhook endpoints for testing.
This Post Has 0 Comments