Make sure you know the difference between PHP sessions and WordPress transients for better data handling and performance in WordPress.
Difference Between PHP explode() and implode() (Simple Explanation)
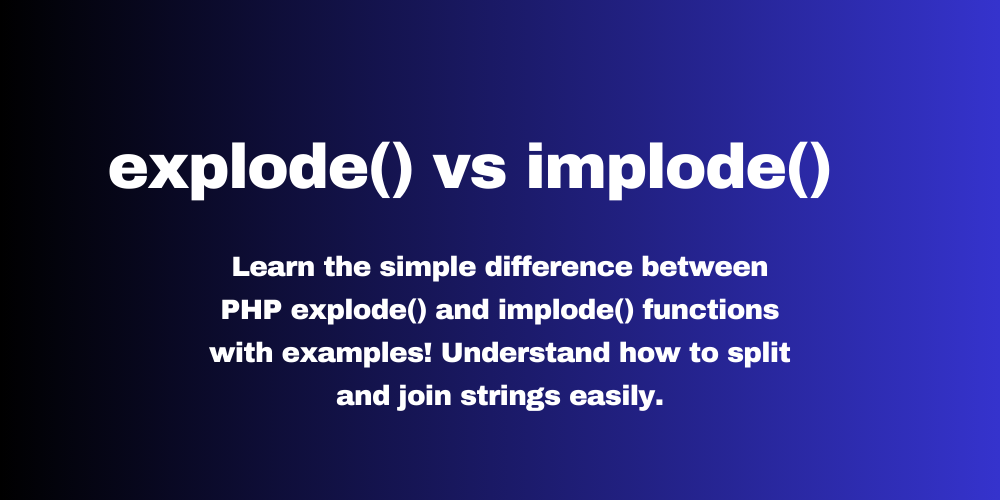
Table of Contents
- Introduction
- What is PHP explode()?
- What is PHP implode()?
- Key Differences Between explode() and implode()
- Examples of explode() and implode()
- When to Use explode() or implode()?
- Conclusion
- FAQs
Introduction
When working with strings in PHP, two common functions you will use are explode()
and implode()
. They sound confusing at first, but they are actually very easy to understand!
This guide will explain the difference between PHP explode() and implode() in simple words.
What is PHP explode()?
The explode()
function in PHP is used to split a string into an array.
Syntax:
explode(separator, string);
separator
: The character where the string will be split.string
: The original string you want to split.
Example:
$string = "apple,banana,orange";
$array = explode(",", $string);
print_r($array);
Output:
Array ( [0] => apple [1] => banana [2] => orange )
What is PHP implode()?
The implode()
function is the opposite of explode()
. It joins array elements into a single string.
Syntax:
implode(separator, array);
separator
: The character that will join the array elements.array
: The array you want to join.
Example:
$array = array("apple", "banana", "orange");
$string = implode("-", $array);
echo $string;
Output:
apple-banana-orange
Key Differences Between explode() and implode()
explode() | implode() |
---|---|
Splits a string into an array | Joins array into a string |
Needs a separator to split | Needs a separator to join |
Example: “a,b,c” → [a, b, c] | Example: [a, b, c] → “a-b-c” |
Examples of explode() and implode()
explode() Example:
$colors = "red,green,blue";
$colorArray = explode(",", $colors);
print_r($colorArray);
implode() Example:
$colors = array("red", "green", "blue");
$colorString = implode(",", $colors);
echo $colorString;
When to Use explode() or implode()?
- Use explode() when you want to break a string into parts.
- Use implode() when you want to combine array items into a string.
Simple Tip:
- explode = split
- implode = join
Conclusion
Now you know the difference between PHP explode() and implode()!
Both functions are very useful when working with strings and arrays. With a little practice, you’ll use them like a pro!
FAQs
Can I use explode() without a separator?
No, you must define a separator when using explode()
.
Can implode() work without specifying a separator?
Yes, if you don’t give a separator, PHP will join elements without any character.
What happens if the separator is not found in the string for explode()?
If the separator is not found, explode()
returns an array with the original string as the only element.
Is there any limit to how many times explode() will split?
Yes, you can provide an optional third parameter to limit how many splits happen.
This Post Has 0 Comments