Learn how to use dynamic pricing in WooCommerce for discounts to boost sales. Step-by-step guide to set rules and offer smart pricing strategies.
How to Dynamically Populate Child ACF Taxonomy Based on Parent Selection
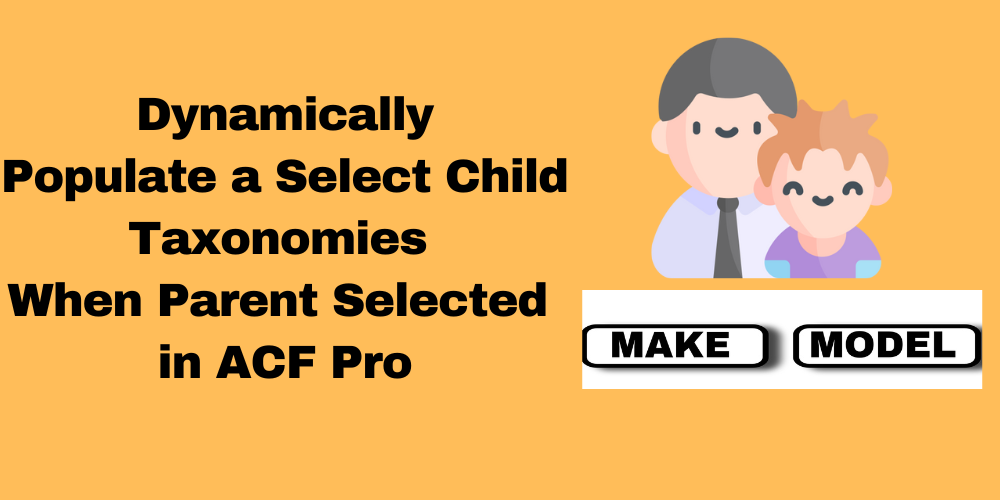
Table of Contents
- Overview
- Setting Up the Custom Taxonomy
- Registering the Taxonomy
- Implementing Parent-Child Relationship ACF Taxonomy
- JavaScript for Dynamic Field Population
- Conclusion
- FAQ
Overview
Taxonomies in WordPress are a powerful way to organize and classify content. However, standard taxonomies may fall short when dealing with complex data structures, such as hierarchical relationships between terms. In this guide, we’ll explore how to implement a dynamic child ACF taxonomy that populates based on the selected parent. This feature improves user experience and streamlines content organization.
Setting Up the Custom Taxonomy
Let’s consider an example where we want to categorize vehicles by their make and model. We’ll create a taxonomy named “Vehicle Models,” where “Make” serves as the parent category and “Model” as the child category.
Step 1: Registering the Taxonomy:
To create the taxonomy, we’ll need to register it in our WordPress theme or plugin using the register_taxonomy()
function.
// Register Custom Taxonomy
function codebykishor_custom_taxonomy() {
$labels = array(
// Add labels here
);
$args = array(
// Add arguments here
);
register_taxonomy( 'vehicle_model', array( 'post' ), $args );
}
add_action( 'init', 'codebykishor_custom_taxonomy', 0 );
Step 2: Implementing Parent-Child Relationship acf taxonomy
Next, we’ll implement the parent-child relationship between the “Make” and “Model” terms within the “Vehicle Models” taxonomy using ACF Pro.
function acf_codebykishor_taxonomy_result( $text, $term, $field, $post_id ) {
if($term->parent != '0'){
$child_term_text="";
$child_term_text .= $term->name;
}
return $child_term_text;
}
add_filter('acf/fields/taxonomy/result/key=field_parent_taxonomy', 'acf_codebykishor_taxonomy_result', 10, 4);
add_filter('acf/fields/taxonomy/result/key=field_parent_child_taxonomy', 'acf_codebykishor_taxonomy_result', 10, 4);
function acf_codebykishor_relation_taxonomy_result( $args, $field, $post_id ){
$parent_id = false;
if ( $field['key'] == 'field_parent_taxonomy' ) { // Parent
$parent_id = 0;
} else if ( $field['key'] == 'field_parent_child_taxonomy' && !empty( $_POST['parent'] ) ) { // Child
$parent_id = (int)$_POST['parent'];
}
if ( $parent_id !== false ) {
$args['parent'] = $parent_id;
}
$args['number'] = 1000;
return $args;
}
add_filter('acf/fields/taxonomy/query/key=field_parent_taxonomy', 'acf_codebykishor_relation_taxonomy_result',10,3); // Parent
add_filter('acf/fields/taxonomy/query/key=field_parent_child_taxonomy', 'acf_codebykishor_relation_taxonomy_result',10,3); // Child
JavaScript for Dynamic Field Population
Lastly, we’ll use JavaScript to dynamically populate the child taxonomy based on the selection of the parent taxonomy.
function acf_car_codebykishor_relation_taxonomy_result_script_footer() {
?>
<script>
(function($){
$(document).ready( function() {
if (typeof acf !== 'undefined') {
acf.add_filter('select2_ajax_data', function( data, args, $input, field, instance ){
var parent_field_key = 'field_parent_taxonomy'; // Parent Field
var target_field_key = 'field_parent_child_taxonomy'; // Child Field
if( data.field_key == target_field_key ){
var field_selector = 'select[name="acf[' + parent_field_key + ']"]'; //the select field holding the values already chosen
if( $(field_selector).val() != '' && $(field_selector).val() != null ){
parent_id = $(field_selector).val();
} else{
parent_id = 0; //nothing chosen yet, offer only top-level terms
}
data.parent = parent_id;
}
return data;
});
};
});
})(jQuery);
</script>
<?php }
add_action('wp_footer', 'acf_car_codebykishor_relation_taxonomy_result_script_footer');
Conclusion:
In this tutorial, we’ve learned how to create a parent-child taxonomy relationship using the Advanced Custom Fields Pro plugin in WordPress. By implementing this technique, you can efficiently organize and categorize your content based on hierarchical classifications.
FAQ
What is the Advanced Custom Fields (ACF) plugin?
A : The ACF plugin allows you to create custom fields for your WordPress site, enhancing your ability to manage and display content beyond the default fields.
What are taxonomies in WordPress?
Answer: Taxonomies are a way to group and categorize content. Common examples include categories and tags.
Can I create multiple levels of hierarchy with ACF Pro?
Answer : Yes, ACF Pro supports multiple levels of hierarchy in taxonomies, allowing for detailed organization.
What is the benefit of using a parent-child taxonomy?
Answer: Parent-child relationships allow for clearer organization of content, making it easier for users to navigate and understand relationships between terms.
How do I display custom taxonomy terms in my templates?
Answer: You can use the get_the_terms() function to retrieve and display custom taxonomy terms associated with a post within your theme templates.
Can I add custom fields to taxonomy terms using ACF?
Answer : Absolutely! ACF lets you add custom fields to taxonomy terms, enabling you to store additional information for each term.
How do I dynamically populate child taxonomies based on parent selections?
Answer : You can use JavaScript in conjunction with ACF’s built-in filters to update child taxonomy options based on the selected parent term.
This Post Has 0 Comments