Learn how to add custom fields to WooCommerce products easily. Follow our step-by-step guide to customize product data on your WooCommerce store.
How to Customize WooCommerce Cart Page with Hooks: Step-by-Step Visual Guide
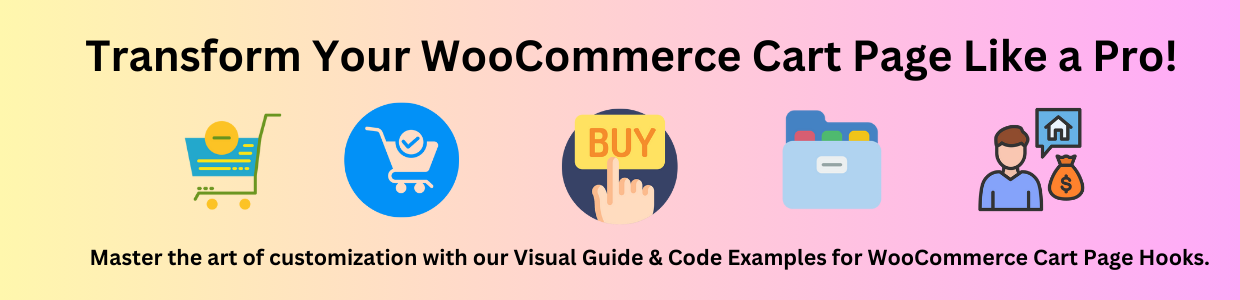
Table of Contents
- Introduction
- What Are WooCommerce Cart Page Hooks?
- WooCommerce Cart Page Visual
- List of WooCommerce Cart Page Hooks
Introduction
When running an eCommerce site using WooCommerce, the cart page plays a vital role in converting visitors into customers. By customizing the WooCommerce cart page hooks, you can enhance the user experience, improve conversion rates, and tailor your online store to fit your specific needs. In this comprehensive guide, we’ll explore WooCommerce cart hooks, their various applications, and how to use them to optimize your cart page design.
If you’ve ever faced issues like the WooCommerce cart page not working or struggled to figure out how to customize the cart page with Elementor, this post is for you. We’ll also cover how to link the cart page in WooCommerce and provide a WooCommerce hooks reference to help you along the way.
What Are WooCommerce Cart Page Hooks?
In WooCommerce, hooks are predefined spots in the code where you can add custom content or functionality. These hooks allow you to insert custom features on the cart page without modifying the core code, making them a powerful tool for developers and store owners alike.
There are several WooCommerce cart page hooks available, and they enable you to customize everything from the cart design to the checkout experience. Understanding these hooks will help you take full control of your cart page’s layout and functionality.

WooCommerce Cart Page
Cart
woocommerce_before_cart
woocommerce_before_cart_table
Product
Price
Quantity
Total
woocommerce_before_cart_contents
woocommerce_cart_contents
woocommerce_cart_coupon
woocommerce_after_cart_contents
woocommerce_before_cart_totals
Cart Totals
Subtotal
$153.00
Shipping
$7.00
Total
$160.00
woocommerce_after_cart_totals
Complete List of WooCommerce Cart Page Hooks
If you’re working with WooCommerce, understanding how to use hooks on the cart page can help you customize the experience for your users. Below is a comprehensive list of essential WooCommerce cart hooks along with examples to assist in your development process
- woocommerce_before_cart
- woocommerce_before_cart_table
- woocommerce_before_cart_contents
- woocommerce_cart_contents
- woocommerce_cart_coupon
- woocommerce_after_cart_contents
- woocommerce_after_cart_table
- woocommerce_cart_collaterals
- woocommerce_before_cart_totals
- woocommerce_cart_totals_before_shipping
- woocommerce_before_shipping_calculator
- woocommerce_after_shipping_calculator
- woocommerce_cart_totals_after_shipping
- woocommerce_cart_totals_before_order_total
- woocommerce_cart_totals_after_order_total
- woocommerce_proceed_to_checkout
- woocommerce_after_cart_totals
- woocommerce_after_cart
Understanding the WooCommerce Cart Page Hooks
To use WooCommerce cart page hooks on your website, you need to add custom code to your theme’s functions.php
file or a custom plugin. These hooks allow you to insert or modify content at specific places on the cart page without altering the core WooCommerce files.
Here’s an explanation of how to use each hook with examples of custom code that you can add to your WordPress site.
1. woocommerce_before_cart
Triggered before the cart page loads.
Example:
add_action('woocommerce_before_cart', 'custom_message_before_cart');
function custom_message_before_cart() {
echo '<p>Welcome to your shopping cart!</p>';
}
2. woocommerce_before_cart_table
This hook is placed before the cart table on the cart page.
Example:
add_action('woocommerce_before_cart_table', 'add_custom_before_cart_table');
function add_custom_before_cart_table() {
echo '<div class="before-cart-table">Custom content before cart table</div>';
}
3. woocommerce_before_cart_contents
Triggered before the cart contents (products) start showing.
Example:
add_action('woocommerce_before_cart_contents', 'before_cart_contents');
function before_cart_contents() {
echo '<p>Items in your cart are listed below:</p>';
}
4. woocommerce_cart_contents
This hook is placed inside the cart table, where the cart items are rendered.
Example:
add_action('woocommerce_cart_contents', 'custom_cart_contents');
function custom_cart_contents() {
echo '<p>We hope you found everything you need!</p>';
}
5. woocommerce_cart_coupon
This hook is placed where the coupon form appears in the cart.
Example:
add_action('woocommerce_cart_coupon', 'add_custom_coupon_field');
function add_custom_coupon_field() {
echo '<p>Enter your coupon code below:</p>';
}
6. woocommerce_after_cart_contents
Triggered after the cart contents.
Example:
add_action('woocommerce_after_cart_contents', 'after_cart_contents');
function after_cart_contents() {
echo '<div>End of the cart contents.</div>';
}
7. woocommerce_after_cart_table
Triggered after the cart table.
Example:
add_action('woocommerce_after_cart_table', 'custom_after_cart_table');
function custom_after_cart_table() {
echo '<div class="after-cart-table">Content after the cart table</div>';
}
8. woocommerce_cart_collaterals
Triggered after the cart table and before the order totals section.
Example:
add_action('woocommerce_cart_collaterals', 'custom_cart_collaterals');
function custom_cart_collaterals() {
echo '<div class="cart-collaterals">Additional information about your cart.</div>';
}
9. woocommerce_before_cart_totals
This hook is triggered before the cart totals are displayed.
Example:
add_action('woocommerce_before_cart_totals', 'before_cart_totals');
function before_cart_totals() {
echo '<div class="before-cart-totals">Cart totals are displayed below</div>';
}
10. woocommerce_cart_totals_before_shipping
Triggered before the shipping section in the cart totals.
Example:
add_action('woocommerce_cart_totals_before_shipping', 'before_shipping_in_cart_totals');
function before_shipping_in_cart_totals() {
echo '<div class="before-shipping">Shipping costs will be calculated based on your location.</div>';
}
11. woocommerce_before_shipping_calculator
This hook is triggered before the shipping calculator form appears.
Example:
add_action('woocommerce_before_shipping_calculator', 'before_shipping_calculator');
function before_shipping_calculator() {
echo '<div class="shipping-calculator">Calculate your shipping below.</div>';
}
12. woocommerce_after_shipping_calculator
Triggered after the shipping calculator form.
Example:
add_action('woocommerce_after_shipping_calculator', 'after_shipping_calculator');
function after_shipping_calculator() {
echo '<p>Your shipping options are calculated above.</p>';
}
13. woocommerce_cart_totals_after_shipping
Triggered after the shipping costs are displayed in the cart totals.
Example:
add_action('woocommerce_cart_totals_after_shipping', 'after_shipping_in_cart_totals');
function after_shipping_in_cart_totals() {
echo '<div class="after-shipping">Additional information after shipping.</div>';
}
14. woocommerce_cart_totals_before_order_total
Triggered just before the order total in the cart totals.
Example:
add_action('woocommerce_cart_totals_before_order_total', 'before_order_total_in_cart_totals');
function before_order_total_in_cart_totals() {
echo '<div class="before-order-total">Summary of your cart before the order total.</div>';
}
15. woocommerce_cart_totals_after_order_total
Triggered after the order total is displayed.
Example:
add_action('woocommerce_cart_totals_after_order_total', 'after_order_total_in_cart_totals');
function after_order_total_in_cart_totals() {
echo '<div class="after-order-total">Additional charges or information after the order total.</div>';
}
16. woocommerce_proceed_to_checkout
This hook appears where the “Proceed to Checkout” button is.
Example:
add_action('woocommerce_proceed_to_checkout', 'custom_proceed_to_checkout');
function custom_proceed_to_checkout() {
echo '<div class="proceed-to-checkout">Ready to checkout? Click below!</div>';
}
17. woocommerce_after_cart_totals
Triggered after the cart totals section.
Example:
add_action('woocommerce_after_cart_totals', 'after_cart_totals');
function after_cart_totals() {
echo '<div class="after-cart-totals">Additional information after the cart totals.</div>';
}
18. woocommerce_after_cart
Triggered after the cart page content is displayed.
Example:
add_action('woocommerce_after_cart', 'custom_content_after_cart');
function custom_content_after_cart() {
echo '<div class="after-cart">Thank you for shopping with us!</div>';
}
Troubleshooting Common Issues: WooCommerce Cart Not Working
If your WooCommerce cart page is not working properly, there are a few common causes to check:
- Plugin Conflicts: Ensure there are no conflicts between your WooCommerce cart plugin and other installed plugins.
- Theme Compatibility: Ensure your theme is fully compatible with WooCommerce. Sometimes, cart page hooks can conflict with custom themes.
- Cache Issues: Clear your website’s cache to ensure the latest updates to your cart page are displayed.
- Outdated WooCommerce Version: Ensure you’re using the latest version of WooCommerce to avoid compatibility issues with cart page hooks.
How to Link Cart Page in WooCommerce
Linking your WooCommerce cart page is essential for directing customers to their cart. You can link it by using the WooCommerce cart URL, which is typically /cart/
. You can also use the WooCommerce cart shortcode [woocommerce_cart]
to embed the cart page anywhere on your site.
Customize Your WooCommerce Cart with Hooks
You can go beyond basic cart functionality with the right WooCommerce cart hooks. For instance, the WooCommerce add to cart hook allows you to modify the behavior of the cart when items are added, while WooCommerce cart filters can adjust cart contents based on customer data.
If you want to change the default behavior, such as redirecting users to the checkout page after adding a product to the cart, you can use the WooCommerce add to cart hook to do so.
Conclusion:
With WooCommerce hooks, you have full control over your cart page’s functionality and design. Whether you are designing a custom shopping cart page using Elementor WooCommerce cart page features, fixing issues like the WooCommerce cart not working, or adding custom content to optimize the shopping experience, cart page hooks WooCommerce are essential.
To make the most of your WooCommerce cart page, refer to the WooCommerce hooks list with example and experiment with different hooks to create a seamless, custom cart experience for your users.
This Post Has 0 Comments