Table of Contents Overview Ultimate Auction Pro Auctions Made Easy for WooCommerce Auctions for WooCommerce…
How to Display a Custom Post List in WordPress (Without Plugins)
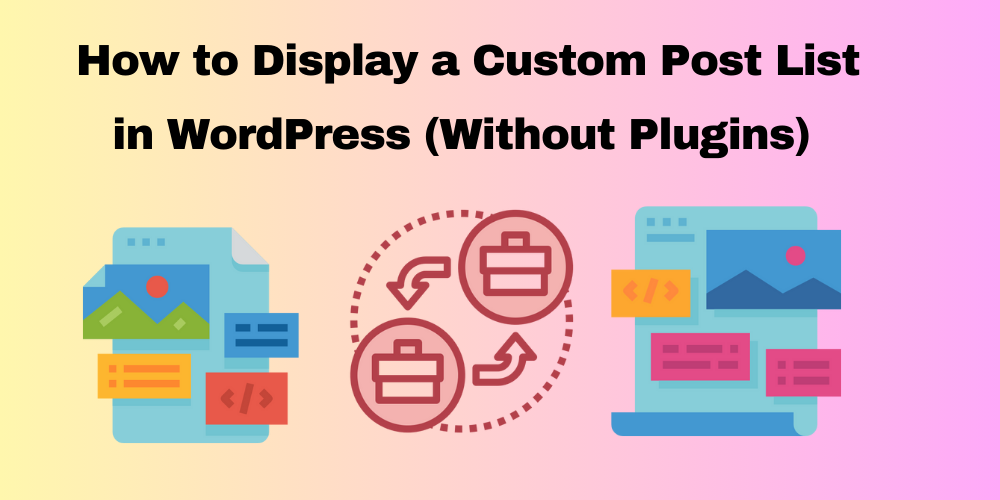
Table of Contents
- Introduction
- Why Avoid Plugins?
- Prepare Your Custom Post Type
- Display Custom Posts with WP_Query
- Customize the Output HTML
- Add the Code to Your Theme
- Style with CSS (Optional)
- Complete Code Example (PHP + HTML + CSS)
- Final Thoughts
- FAQs
1. Introduction
Do you want to display a custom post list in WordPress without plugins? You’re in the right place! This guide will help you do that with a few lines of code. No need to install extra plugins. It’s clean, fast, and lightweight.
2. Why Avoid Plugins?
Plugins are helpful, but using too many can slow down your website. If you only need a simple post list, make sure to do it with code to improve performance and reduce maintenance.
3. Prepare Your Custom Post Type
If you haven’t already created a custom post type (CPT), use the following code in your theme’s functions.php
:
function create_custom_post_type() {
register_post_type('books',
array(
'labels' => array(
'name' => __('Books'),
'singular_name' => __('Book')
),
'public' => true,
'has_archive' => true,
'rewrite' => array('slug' => 'books'),
'supports' => array('title', 'editor', 'thumbnail')
)
);
}
add_action('init', 'create_custom_post_type');
4. Display Custom Posts with WP_Query
To display the post list, use WP_Query
in your theme’s template file (like page.php
, single.php
, or a custom template):
<?php
$args = array(
'post_type' => 'books',
'posts_per_page' => 5
);
$custom_query = new WP_Query($args);
if ($custom_query->have_posts()) :
echo '<ul class="custom-post-list">';
while ($custom_query->have_posts()) : $custom_query->the_post();
echo '<li><a href="' . get_permalink() . '">' . get_the_title() . '</a></li>';
endwhile;
echo '</ul>';
wp_reset_postdata();
else :
echo '<p>No posts found.</p>';
endif;
?>
5. Customize the Output HTML
You can customize the list by adding images, excerpts, or any other field:
echo '<li>';
the_post_thumbnail('thumbnail');
echo '<a href="' . get_permalink() . '">' . get_the_title() . '</a>';
echo '<p>' . get_the_excerpt() . '</p>';
echo '</li>';
6. Add the Code to Your Theme
Add the above code to a custom template or existing page template. You can even create a new page template like this:
<?php
/*
Template Name: Custom Post List
*/
get_header();
// Insert the WP_Query code here
get_footer();
?>
Then assign this template to a page from the WordPress admin.
7. Style with CSS (Optional)
Use CSS to style your custom post list:
.custom-post-list {
list-style: none;
padding: 0;
}
.custom-post-list li {
margin-bottom: 20px;
}
.custom-post-list img {
max-width: 100px;
display: block;
}
Complete Code Example (PHP + HTML + CSS)
Below is the full working example of how to display a custom post list in WordPress without plugins. This includes both the PHP for querying the posts and the CSS for basic styling.
Template Code (Paste into a custom page template or inside page.php
)
<?php
/*
Template Name: Custom Post List
*/
get_header(); ?>
<h2>Custom Post List</h2>
<?php
$args = array(
'post_type' => 'books', // Change this to your custom post type slug
'posts_per_page' => 5
);
$custom_query = new WP_Query($args);
if ($custom_query->have_posts()) :
echo '<ul class="custom-post-list">';
while ($custom_query->have_posts()) : $custom_query->the_post(); ?>
<li>
<?php if (has_post_thumbnail()) : ?>
<div class="custom-thumb"><?php the_post_thumbnail('thumbnail'); ?></div>
<?php endif; ?>
<a href="<?php the_permalink(); ?>"><?php the_title(); ?></a>
<p><?php echo get_the_excerpt(); ?></p>
</li>
<?php endwhile;
echo '</ul>';
wp_reset_postdata();
else :
echo '<p>No posts found.</p>';
endif;
?>
<?php get_footer(); ?>
CSS (Paste into your style.css
)
.custom-post-list {
list-style: none;
padding: 0;
margin: 0;
}
.custom-post-list li {
margin-bottom: 30px;
border-bottom: 1px solid #eee;
padding-bottom: 20px;
}
.custom-post-list a {
font-size: 18px;
font-weight: bold;
color: #333;
text-decoration: none;
}
.custom-post-list a:hover {
text-decoration: underline;
}
.custom-thumb {
margin-bottom: 10px;
}
.custom-thumb img {
max-width: 100px;
height: auto;
display: block;
}
Now, users just need to:
- Create a page in WordPress.
- Assign it the “Custom Post List” template.
- Make sure their custom post type (
books
in this case) exists. - Save and view the page — your post list will appear!
Let me know if you want me to include pagination, filter by category, or convert this into a downloadable file!
8. Final Thoughts
If you want full control over how your post lists look and function, skip the plugins. Use simple code to make sure your website runs faster and cleaner. This is a great solution for developers and site owners who want performance and flexibility.
9. FAQs
Can I use this method for any post type in WordPress?
Yes, you can use this method for any post type. Just replace 'books'
in the code with your desired custom post type slug, like 'projects'
, 'events'
, or 'portfolio'
.
Make sure the post type is already registered in your WordPress site.
Where should I place the custom post list code?
You should use a WordPress child theme to avoid losing changes during theme updates. Place the code in templates like page.php
, single.php
, or better, create a custom page template.
Make sure to back up your theme before editing files.
Will this custom post list work with all WordPress themes?
Yes, this method works with most themes that follow standard WordPress coding practices.
To keep your site safe and stable, always use a child theme and make sure your theme supports custom templates.
How can I display posts by category or tag?
You can filter your custom post list using WordPress query arguments. Add this inside your $args
array:
For tags: 'tag' => 'featured'
This will make sure only posts from that category or tag are shown.
For categories: 'category_name' => 'news'
This Post Has 0 Comments