Overview The General Data Protection Regulation (GDPR) requires websites to protect their users' data. If…
How to Fetch Data into a Custom WordPress Table Using WP_List_Table
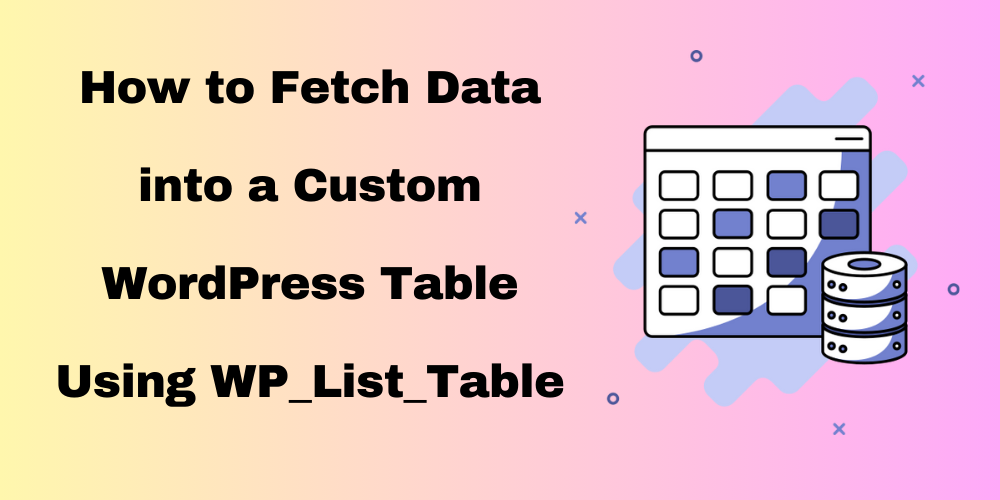
Overview
If you want to display custom data in WordPress, using WP_List_Table
is a great option. This guide will show you how to fetch and display data from a custom table in a WordPress admin page. Let’s get started!
Create Your Custom Table
First, you need a custom database table. Use the following SQL query to create one:
CREATE TABLE wp_custom_table (
id mediumint(9) NOT NULL AUTO_INCREMENT,
name varchar(100) NOT NULL,
email varchar(100) NOT NULL,
PRIMARY KEY (id)
);
You can run this in your database management tool (like phpMyAdmin).
Create Your Custom Class
Next, create a custom class that extends WP_List_Table
. This class will handle displaying your data.
//code for Fetch Data into a Custom WordPress Table
if (!class_exists('WP_List_Table')) {
require_once(ABSPATH . 'wp-admin/includes/class-wp-list-table.php');
}
class Custom_Table extends WP_List_Table {
function column_default($item, $column_name) {
return $item[$column_name];
}
function get_columns() {
return [
'id' => 'ID',
'name' => 'Name',
'email' => 'Email',
];
}
function prepare_items() {
global $wpdb;
$table_name = $wpdb->prefix . 'custom_table';
$this->items = $wpdb->get_results("SELECT * FROM $table_name", ARRAY_A);
}
}
Display the Table
Now, you need to add your table to the admin area. Use the following code in your plugin or theme’s functions.php file.
function my_custom_table_page() {
$customTable = new Custom_Table();
$customTable->prepare_items();
?>
<div class="wrap">
<h2>Custom Table</h2>
<form method="post">
<?php $customTable->display(); ?>
</form>
</div>
<?php
}
function my_custom_menu() {
add_menu_page('Custom Table', 'Custom Table', 'manage_options', 'custom-table', 'my_custom_table_page');
}
add_action('admin_menu', 'my_custom_menu');
Style Your Table
You might want to style your table for better user experience. Add custom CSS to your admin area by enqueuing styles.
function my_custom_table_styles() {
echo '<style>
.wp-list-table { border-collapse: collapse; width: 100%; }
.wp-list-table th, .wp-list-table td { padding: 10px; border: 1px solid #ddd; }
</style>';
}
add_action('admin_head', 'my_custom_table_styles');
Complete Code
Here’s the complete code for fetching data into a custom WordPress table using WP_List_Table
, all in one place:
<?php
// Include this code in your plugin or theme's functions.php file
// Step 1: Create Custom Table (Run this in your database)
function create_custom_table() {
global $wpdb;
$table_name = $wpdb->prefix . 'custom_table';
$charset_collate = $wpdb->get_charset_collate();
$sql = "CREATE TABLE $table_name (
id mediumint(9) NOT NULL AUTO_INCREMENT,
name varchar(100) NOT NULL,
email varchar(100) NOT NULL,
PRIMARY KEY (id)
) $charset_collate;";
require_once(ABSPATH . 'wp-admin/includes/upgrade.php');
dbDelta($sql);
}
// Uncomment to create table (run once)
// add_action('after_switch_theme', 'create_custom_table');
// Step 2: Create Custom Class
if (!class_exists('WP_List_Table')) {
require_once(ABSPATH . 'wp-admin/includes/class-wp-list-table.php');
}
class Custom_Table extends WP_List_Table {
function column_default($item, $column_name) {
return $item[$column_name];
}
function get_columns() {
return [
'id' => 'ID',
'name' => 'Name',
'email' => 'Email',
];
}
function prepare_items() {
global $wpdb;
$table_name = $wpdb->prefix . 'custom_table';
$this->items = $wpdb->get_results("SELECT * FROM $table_name", ARRAY_A);
}
}
// Step 3: Display the Table
function my_custom_table_page() {
$customTable = new Custom_Table();
$customTable->prepare_items();
?>
<div class="wrap">
<h2>Custom Table</h2>
<form method="post">
<?php $customTable->display(); ?>
</form>
</div>
<?php
}
// Step 4: Add Menu Item
function my_custom_menu() {
add_menu_page('Custom Table', 'Custom Table', 'manage_options', 'custom-table', 'my_custom_table_page');
}
add_action('admin_menu', 'my_custom_menu');
// Step 5: Style Your Table
function my_custom_table_styles() {
echo '<style>
.wp-list-table { border-collapse: collapse; width: 100%; }
.wp-list-table th, .wp-list-table td { padding: 10px; border: 1px solid #ddd; }
</style>';
}
add_action('admin_head', 'my_custom_table_styles');
?>
Simple WordPress Plugin
Here’s how to create a simple WordPress plugin to fetch data into a custom table using WP_List_Table
. Follow these steps:
Step 1: Create the Plugin File
- Create a Folder: Go to your WordPress installation directory and navigate to
wp-content/plugins
. Create a new folder calledcustom-table-plugin
. - Create the Plugin File: Inside the
custom-table-plugin
folder, create a file namedcustom-table-plugin.php
.
Step 2: Add the Plugin Code
Open custom-table-plugin.php
and add the following code:
<?php
/**
* Plugin Name: Custom Table Plugin
* Description: A simple plugin to display a custom table using WP_List_Table.
* Version: 1.0
* Author: Your Name
*/
// Exit if accessed directly
if (!defined('ABSPATH')) {
exit;
}
// Step 1: Create Custom Table
function create_custom_table() {
global $wpdb;
$table_name = $wpdb->prefix . 'custom_table';
$charset_collate = $wpdb->get_charset_collate();
$sql = "CREATE TABLE $table_name (
id mediumint(9) NOT NULL AUTO_INCREMENT,
name varchar(100) NOT NULL,
email varchar(100) NOT NULL,
PRIMARY KEY (id)
) $charset_collate;";
require_once(ABSPATH . 'wp-admin/includes/upgrade.php');
dbDelta($sql);
}
// Step 2: Run the table creation on plugin activation
register_activation_hook(__FILE__, 'create_custom_table');
// Step 3: Create Custom Class
if (!class_exists('WP_List_Table')) {
require_once(ABSPATH . 'wp-admin/includes/class-wp-list-table.php');
}
class Custom_Table extends WP_List_Table {
function column_default($item, $column_name) {
return $item[$column_name];
}
function get_columns() {
return [
'id' => 'ID',
'name' => 'Name',
'email' => 'Email',
];
}
function prepare_items() {
global $wpdb;
$table_name = $wpdb->prefix . 'custom_table';
$this->items = $wpdb->get_results("SELECT * FROM $table_name", ARRAY_A);
}
}
// Step 4: Display the Table
function my_custom_table_page() {
$customTable = new Custom_Table();
$customTable->prepare_items();
?>
<div class="wrap">
<h2>Custom Table</h2>
<form method="post">
<?php $customTable->display(); ?>
</form>
</div>
<?php
}
// Step 5: Add Menu Item
function my_custom_menu() {
add_menu_page('Custom Table', 'Custom Table', 'manage_options', 'custom-table', 'my_custom_table_page');
}
add_action('admin_menu', 'my_custom_menu');
// Step 6: Style Your Table
function my_custom_table_styles() {
echo '<style>
.wp-list-table { border-collapse: collapse; width: 100%; }
.wp-list-table th, .wp-list-table td { padding: 10px; border: 1px solid #ddd; }
</style>';
}
add_action('admin_head', 'my_custom_table_styles');
Step 3: Install the Plugin
- Upload the Plugin: Go to your WordPress admin dashboard, navigate to Plugins > Add New, then click on Upload Plugin. Upload the
custom-table-plugin
folder as a zip file or upload it via FTP to thewp-content/plugins
directory. - Activate the Plugin: Once uploaded, find the “Custom Table Plugin” in your plugins list and click Activate.
Step 4: View Your Table
After activation, you’ll see a new menu item labeled “Custom Table” in your WordPress admin sidebar. Click on it to view your custom table populated with data from the database.
Conclusion
Using WP_List_Table
to fetch and display data from a custom WordPress table is a straightforward process. This method gives you a flexible way to manage and present data within your WordPress site. You can expand on this by adding functionalities like data entry forms or filters.
Feel free to customize this plugin further to meet your needs! If you have any questions or need assistance, don’t hesitate to reach out.
FAQ
What is WP_List_Table
?
Class for displaying tabular data in the WordPress admin area.
How do I create the custom table?
Run the provided SQL query in your database management tool.
Can I add more columns?
Yes, modify the get_columns()
method in the Custom_Table
class.
How to fetch data from multiple tables?
Adjust the SQL query in the prepare_items()
method.
Is database manipulation safe?
Use WordPress functions like wpdb->prepare()
to ensure safety.
This Post Has 0 Comments