Table of Contents Overview Ultimate Auction Pro Auctions Made Easy for WooCommerce Auctions for WooCommerce…
What are WordPress Hooks and How to Use Them Effectively
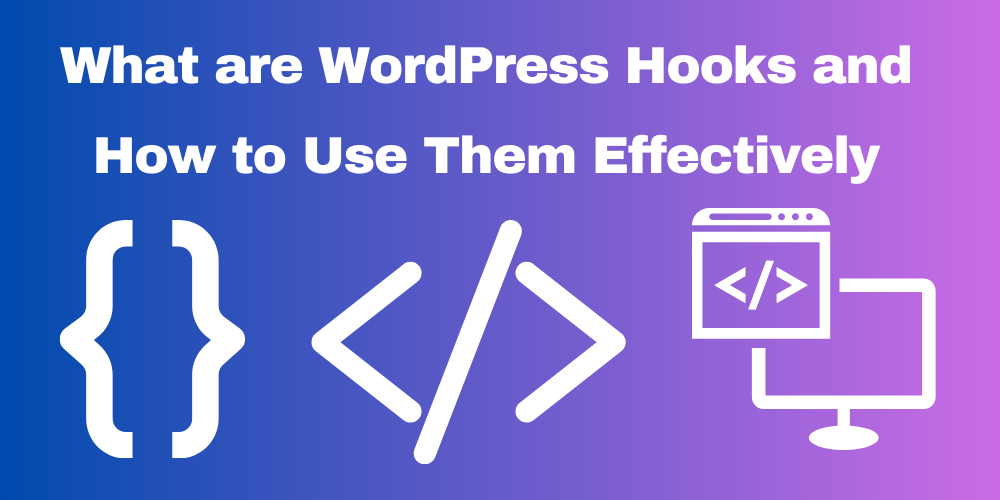
Table of Contents
- What is a Hook in WordPress?
- Types of Hooks in WordPress
- Action and Filter Hooks: Key Differences
- How to Use Hooks in WordPress
- How to Add Action Hooks in WordPress
- How to Create Custom Hooks in WordPress
- WooCommerce Hooks in WordPress
- Commonly Used Hooks in WordPress
- The Role of Hooks and Filters in WordPress
- Conclusion
- FAQ
Overview
WordPress is a versatile platform for building websites and blogs. One of its most powerful features is the use of hooks. But what exactly are hooks in WordPress, and how can you leverage them for custom functionality? In this blog, we’ll dive into the details of WordPress hooks, types of hooks in WordPress, and how to use them to enhance your site.
What is a Hook in WordPress?
A hook in WordPress is a way for developers to modify or extend the functionality of WordPress without changing the core files. Hooks allow you to add custom code at specific points during the WordPress page load process. They make WordPress highly flexible, enabling you to customize your site without altering the core structure.
There are two main types of hooks in WordPress:
- Action Hooks
- Filter Hooks
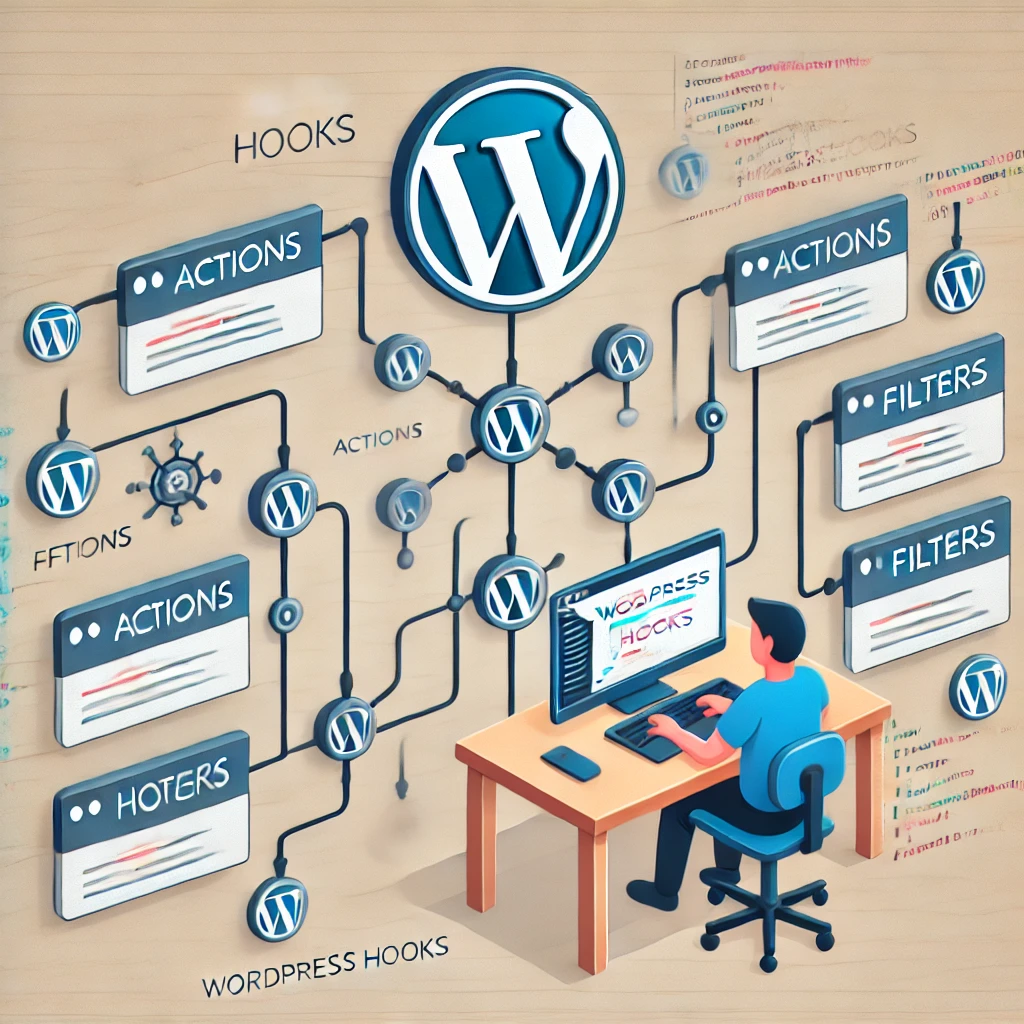
Types of Hooks in WordPress
Action Hooks in WordPress
Action hooks in WordPress are used to perform specific actions at various points in the WordPress execution process. When WordPress triggers an action hook, the code you attach to that hook runs. For example, you might use action hooks to add custom HTML, perform database operations, or include other functionality.
Example of Action Hooks in WordPress
add_action('wp_footer', 'my_custom_footer_code');
function my_custom_footer_code() {
echo '<p>Custom footer content here!</p>';
}
In this example, wp_footer
is an action hook, and we’ve attached a custom function my_custom_footer_code()
to it. When the footer is loaded, our custom content will be displayed.
Filter Hooks in WordPress
Filter hooks allow you to modify data before it is used or displayed. For example, you can modify the content of a post before it is shown on the front end or change the output of a function.
Example of Filter Hooks in WordPress
add_filter('the_content', 'custom_content_filter');
function custom_content_filter($content) {
return $content . '<p>Custom content appended to the post.</p>';
}
Here, the the_content
filter hook is used to append custom content at the end of the post.
Action and Filter Hooks in WordPress: How They Differ
- Action hooks trigger actions at specific points but do not modify data directly.
- Filter hooks allow for modifications to data, giving you the ability to manipulate or enhance content or behavior.
Both types of hooks serve distinct but complementary purposes in WordPress development.
Difference Between Filter and Action Hooks?
Feature | Action Hooks | Filter Hooks |
---|---|---|
Purpose | Trigger actions at specific points in the WordPress lifecycle. | Modify or filter data before it’s used or displayed. |
Main Use | Perform actions such as adding content, performing calculations, etc. | Modify content or behavior (e.g., change text or output). |
Return Value | No return value is required. Action hooks perform actions. | Requires a return value; the filtered data is returned. |
Function Example | add_action('wp_footer', 'custom_footer_function') | add_filter('the_content', 'modify_post_content') |
Where Used | Used to add or modify functionality in different areas of WordPress. | Used to modify data like content, settings, or options. |
Examples of Common Hooks | wp_head , wp_footer , init , admin_menu | the_content , the_title , widget_text , the_excerpt |
Execution Timing | Action hooks are executed at the specified time (e.g., page load, admin menu creation). | Filter hooks are executed when data is passed through them, modifying it before display or processing. |
Modification of Data | Does not modify data, only triggers custom code. | Modifies or filters data before it is saved or displayed. |
Use Case | Adding HTML, CSS, or scripts, or running processes. | Changing or filtering content, such as modifying post text or changing output. |
How to Use Hooks in WordPress
Using hooks in WordPress is simple. You can add hooks in your theme’s functions.php file or a custom plugin. By using the add_action()
or add_filter()
functions, you can attach your custom functions to predefined hooks in WordPress.
For example, if you want to add a custom message to the WordPress login screen, you can use an action hook like this:
add_action('login_message', 'custom_login_message');
function custom_login_message($message) {
return $message . '<p>Welcome to My Custom WordPress Site!</p>';
}
This will display the custom message on the login page using the login_message
action hook.
How to Add Action Hooks in WordPress
You can add action hooks to various points of the WordPress lifecycle. Here are some commonly used action hooks in WordPress:
- wp_head – Used to add custom scripts or styles in the
<head>
section. - wp_footer – Adds content to the footer area of the site.
- init – Runs after WordPress has loaded but before any headers are sent.
- admin_menu – Adds custom admin menu items.
Types of Action Hooks in WordPress
Action hooks come in different forms, such as early and late hooks. Understanding the hook priority allows you to control the order in which actions are executed.
How to Create Custom Hooks in WordPress
Sometimes, you may want to create your own hooks to provide flexibility in your theme or plugin. Here’s a simple example of creating a custom hook in WordPress:
do_action('my_custom_hook');
function custom_hook_function() {
echo 'This is a custom hook!';
}
add_action('my_custom_hook', 'custom_hook_function');
This example shows how to create a custom hook called my_custom_hook
and attach a custom function to it. Whenever do_action('my_custom_hook')
is triggered, the custom_hook_function()
will run.
How to Create Custom Filter Hooks in WordPress
Just like action hooks, you can also create custom filter hooks to modify data in a flexible way within your theme or plugin. Creating custom filters can be useful when you need to alter content before it’s displayed or saved.
Here’s a simple example of how to create and use a custom filter in WordPress:
Example: Creating and Using a Custom Filter Hook
Create the Custom Filter Hook
To create a custom filter hook, you’ll use the apply_filters()
function. This allows other functions to modify the data passed to it.
// Create the custom filter hook
$content = apply_filters('my_custom_filter', $content);
Add a Function to the Custom Filter Hook
Next, you can attach a function to this custom filter using add_filter()
. This function will modify the data before it’s used.
// Define the function that will modify the content
function modify_custom_content($content) {
$content .= ' This is custom filtered content.';
return $content; // Return the modified content
}
// Attach the function to the custom filter hook
add_filter('my_custom_filter', 'modify_custom_content');
Using the Custom Filter
Whenever you use apply_filters('my_custom_filter', $content)
, the content will be passed through any functions attached to this filter hook, and they will modify the content before it’s returned.
// Example of using the custom filter
$content = "Hello, this is the original content.";
$content = apply_filters('my_custom_filter', $content); // Apply custom filter
echo $content; // Output: "Hello, this is the original content. This is custom filtered content."
How it Works:
- The
apply_filters()
function is used to declare where the filter should be applied. - The
add_filter()
function hooks your custom function to the filter, modifying the data (in this case,$content
) before it’s used. - You can create as many custom filters as needed, depending on the functionality you want to modify.
WooCommerce Hooks in WordPress
If you’re working with WooCommerce, you’ll be pleased to know that it also makes extensive use of hooks. WooCommerce hooks allow you to add or modify functionality in the shop, checkout, and product pages.
For example, you can add content to the WooCommerce product pages using:
add_action('woocommerce_after_single_product', 'custom_product_content');
function custom_product_content() {
echo '<p>Custom content below the product.</p>';
}
This hook adds custom content after the product on a WooCommerce single product page.
List of Hooks in WordPress
There are many hooks in WordPress that allow you to modify the behavior and appearance of your site. Here’s a brief list of commonly used hooks:
- wp_head – Output custom code in the
<head>
section. - wp_footer – Add custom content to the footer.
- the_content – Modify post content.
- admin_menu – Modify the WordPress admin menu.
- login_form – Modify the login form.
For a complete list, check out the WordPress Codex or the WordPress Plugin Developer Handbook.
The Role of Hooks and Filters in WordPress
Both hooks and filters are integral to WordPress development, allowing developers to:
- Customize content and extend functionality without modifying core files.
- Modify data before it’s displayed or saved, giving you more control over how content is presented.
By understanding how to use action hooks and filter hooks effectively, you can greatly enhance the flexibility and functionality of your WordPress site.
Conclusion
Hooks are a powerful concept in WordPress that allows you to customize your site without touching core files. Whether you’re using action hooks, filter hooks, or WooCommerce hooks, learning how to properly use hooks can significantly improve your site’s functionality.
By following the examples provided in this guide, you can start implementing hooks in WordPress today. Whether you’re a beginner or an advanced developer, hooks in WordPress provide endless possibilities for customization.
FAQ
What are WordPress hooks?
WordPress hooks are special points in the WordPress code where developers can add custom functions or modify behavior. They allow you to extend and customize WordPress without altering core files.
What is the difference between action hooks and filter hooks in WordPress?
Action hooks are used to perform actions at specific points in WordPress, such as adding content or running processes.
Filter hooks are used to modify data before it’s displayed, like adjusting post content or output.
How do I create custom hooks in WordPress?
To create a custom hook in WordPress, use do_action()
for action hooks and apply_filters()
for filter hooks. This lets you define custom functionality or modify data at specific points.
How do I use action hooks in WordPress?
To use action hooks, attach a custom function to a hook using the add_action()
function. For example, you can add custom content to the footer with the wp_footer
action hook.
How do I use filter hooks in WordPress?
Use add_filter()
to attach a function that modifies content before it’s displayed. For instance, the the_content
filter hook can modify the content of WordPress posts.
Where should I add hooks in WordPress?
You can add hooks in your theme’s functions.php
file or in a custom plugin. This allows you to extend functionality and modify content wherever needed.
This Post Has 0 Comments