Learn the difference between free and premium WordPress themes. Find out which one is right for your website with pros, cons, and tips.
What is the Difference Between Session and Cookie in PHP?
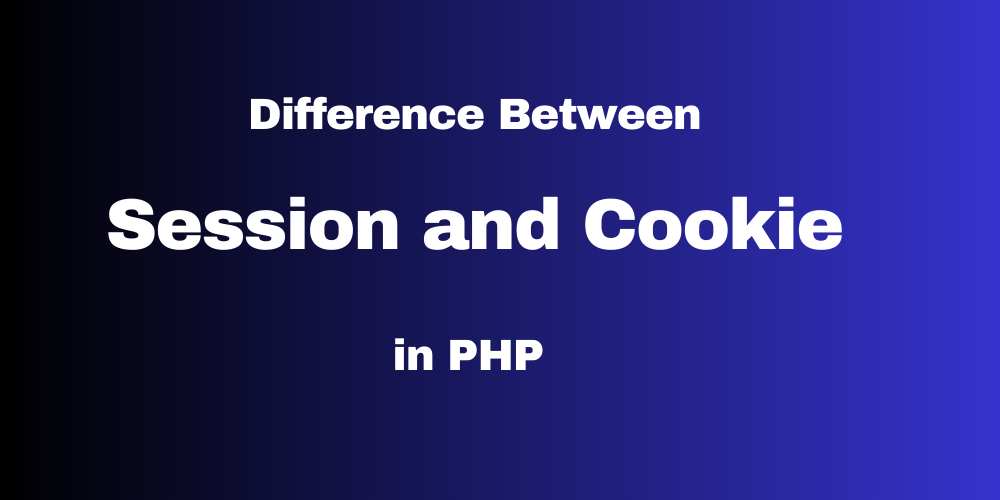
In PHP, both sessions and cookies are used to store user data. But they work in different ways. In this guide, you’ll learn what they are, how they work, real examples, and when to use each one.
Table of Contents
- What is a Cookie in PHP?
- What is a Session in PHP?
- Real Example: When to Use Cookie
- Real Example: When to Use Session
- Key Differences Between Session and Cookie
- Advantages and Disadvantages
- When to Use a Cookie or Session?
- Example Code: Cookie vs Session
- Conclusion
- FAQ
What is a Cookie in PHP?
A cookie is a small file that is stored on the user’s browser. It can store information like username, preferences, or last visit time.
How it works:
- Created using
setcookie()
function. - Stored in the user’s browser.
- Sent with every request to the server.
- Can be set to expire at a specific time.
Example:
setcookie("username", "TeckyKP", time() + 3600); // expires in 1 hour
What is a Session in PHP?
A session stores information on the server. It uses a unique ID stored in the user’s browser to link the session data.
How it works:
- Starts with
session_start()
. - Data is stored on the server.
- Session ID is stored in a cookie or URL.
- Ends when the browser is closed or session expires.
Example:
session_start();
$_SESSION["username"] = "TeckyKP";
Real Example: When to Use Cookie
Use Case: Remember User’s Preferred Theme
// Set cookie for dark mode
setcookie("theme", "dark", time() + (86400 * 30)); // 30 days
// Read cookie
if(isset($_COOKIE["theme"])) {
echo "Theme: " . $_COOKIE["theme"];
} else {
echo "Default theme";
}
Why? It’s non-sensitive info and should stay after closing the browser.
Real Example: When to Use Session
Use Case: Store Logged-in User Info
// Set session
session_start();
$_SESSION["user"] = "TeckyKP";
// Access session
session_start();
if(isset($_SESSION["user"])) {
echo "Welcome, " . $_SESSION["user"];
} else {
echo "Please log in.";
}
Why? Login info is sensitive and should be kept on the server.
Key Differences Between Session and Cookie
Feature | Cookie | Session |
---|---|---|
Storage | User’s browser | Web server |
Expiry | Set manually | Ends with session/browser |
Security | Less secure (client-side) | More secure (server-side) |
Data size limit | Around 4KB | No specific limit |
Use case | Remember preferences | Store login/session data |
Advantages and Disadvantages
✅ Cookies – Advantages
- Store data even after browser is closed.
- Good for non-sensitive preferences (e.g., theme).
- Light on server.
❌ Cookies – Disadvantages
- Can be viewed/changed by users.
- Limited size (~4KB).
- Not safe for passwords or sensitive info.
✅ Sessions – Advantages
- Secure (server-side storage).
- No data size limit.
- Ideal for login, cart, etc.
❌ Sessions – Disadvantages
- Data lost when browser closes.
- Uses more server memory.
- Needs
session_start()
on every page.
When to Use a Cookie or Session?
Scenario | Use Cookie? | Use Session? |
Remember user theme or language | ✅ Yes | ❌ No |
Store user login info | ❌ No | ✅ Yes |
Save cart items during checkout | ❌ No | ✅ Yes |
Track recently visited products | ✅ Yes | ❌ No |
Example Code: Cookie vs Session
Set a Cookie:
setcookie("user", "TeckyKP", time() + 86400); // 1 day
Set a Session:
session_start();
$_SESSION["user"] = "TeckyKP";
Conclusion
The main difference between session and cookie in PHP is where data is stored. Cookies store data in the browser. Sessions store data on the server. Use cookies for light, long-term data. Use sessions for secure, short-term data.
FAQ
What is more secure, session or cookie?
Session is more secure because data is stored on the server.
Can I use both session and cookie together?
Yes, many websites use both for different needs.
Does a cookie work if the browser is closed?
Yes, if you set an expiry time.
Can cookies store passwords?
No, it’s not safe. Use sessions for login info.
How long does a PHP session last?
Until the browser is closed or the session times out (usually 24 minutes).
This Post Has 0 Comments