Table of Contents Best WooCommerce Product Filter Plugins Why Use WooCommerce Product Filters? Benefits of…
How to Add a Product Programmatically in WooCommerce: Step-by-Step Guide
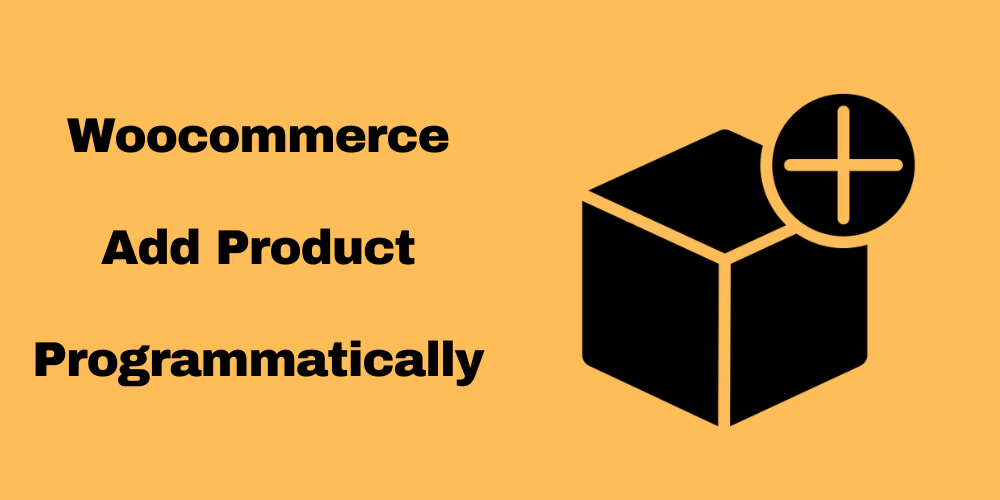
Table of Contents
- Overview
- Prerequisites
- Why Add Products Programmatically?
- Step-by-Step Guide
- Customize Your Product
- Conclusion
- FAQ
Overview
In the world of eCommerce, managing products efficiently is crucial for success. WooCommerce, a popular WordPress plugin, provides a robust API that allows developers to programmatically add products to their online stores. In this post, we will explore how to achieve this through a straightforward example of adding a product programmatically in WooCommerce.
Prerequisites
Before we dive in, ensure you have the following:
- A WordPress site with WooCommerce installed and activated.
- Basic knowledge of PHP and WordPress development.
- Access to your theme’s
functions.php
file or a custom plugin.
Why Add Products Programmatically?
Adding products manually can be time-consuming. For businesses with large inventories, this approach is inefficient. Therefore, programmatically adding products helps automate the process, making it faster and easier.
Step-by-Step Guide
To start, open your theme’s functions.php
file or create a custom plugin to add your code.
WooCommerce provides a WC_Product
class that simplifies the creation of new products. Below is a basic example:
// Function to add a product programmatically
function add_custom_product() {
// Check if function exists to avoid errors
if ( ! class_exists( 'WC_Product' ) ) {
return;
}
// Set up product data
$product = new WC_Product();
// Set product properties
$product->set_name( 'Sample Product' ); // Product name
$product->set_regular_price( '29.99' ); // Regular price
$product->set_description( 'This is a sample product description.' ); // Product description
$product->set_short_description( 'Short description for the sample product.' ); // Short description
$product->set_sku( 'SP-001' ); // SKU (Stock Keeping Unit)
$product->set_manage_stock( true ); // Enable stock management
$product->set_stock_quantity( 100 ); // Set stock quantity
$product->set_category_ids( array( 15 ) ); // Set category ID (replace with your category ID)
// Add product to WooCommerce
$product_id = $product->save();
if ( $product_id ) {
echo 'Product added successfully with ID: ' . $product_id;
} else {
echo 'Failed to add product.';
}
}
// Hook the function into 'init' action
add_action( 'init', 'add_custom_product' );
Customize Your Product
You can further customize your product by adding attributes, images, and more. For example, here’s how to set a product image and add product attributes:
// Set product image
$product->set_image_id( 123 ); // Replace 123 with your attachment ID
// Add attributes
$attributes = array(
'color' => array(
'name' => 'Color',
'value' => 'Red',
'position' => 0,
'is_visible' => 1,
'is_variation' => 0,
'is_taxonomy' => 0,
),
);
$product->set_attributes( $attributes );
Once you’ve added your code, refresh your WordPress site. If everything is set up correctly, you should see a message indicating that the product has been added successfully.
Conclusion
Adding products programmatically in WooCommerce can save you time and effort, especially for larger catalogs. By utilizing the WooCommerce API, you can manage your products in bulk more efficiently. Experiment with different product types, attributes, and settings to suit your needs.
FAQ
What if I encounter errors when adding products?
Ensure that WooCommerce is installed and activated. Check your PHP code for syntax errors.
Can I add variable products programmatically?
Yes, you can create variable products by using the WC_Product_Variable
class and setting variations accordingly.
How do I find the attachment ID for product images?
Upload an image to your media library, click on it, and find the ID in the URL.
Is it safe to add products via code?
Yes, as long as you test the code on a staging site first to avoid issues on your live site.
This Post Has 0 Comments